Animation In Flutter
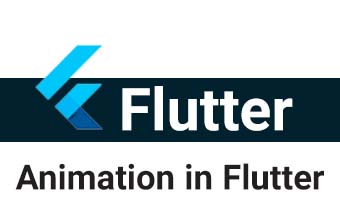
مبحث انیمیش در فلاتر ، از جذاب ترین مباحث کار می باشد . شما به راحتی می توانید افکت های حرکتی مختلفی را به ویجت های موجود در UI بدهید . در زیر نمونه ای کوچک از پیاده سازی یک انیمیشن قرار دارد . در این مثال سعی شده روش کار با چهار جزء سازنده یک انیمیشن شامل : Animation , AnimationController , Tween , AnimatedBuilder به نمایش گذاشته شود .
کد پیاده سازی این انیمیشن به شکل زیر است :
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( debugShowCheckedModeBanner: false, home: MyApp(), )); } class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> with SingleTickerProviderStateMixin { AnimationController controller; // تعریف یک انیمیشن کنترلر Animation<double> animation; // تعریف یک انیمیشن @override void initState() { // TODO: implement initState super.initState(); // مقداردهی به کنترلر controller = AnimationController( vsync: this, duration: Duration(milliseconds: 2000)); // مقداردهی به انیمیشن animation = Tween(begin: 0.0, end: 300.0) .animate(CurvedAnimation(parent: controller, curve: Curves.bounceOut)); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('انیمیشن در فلاتر'), centerTitle: true, ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ // استفاده از انیمیش بیلدر جهت ساخت انیمیشن AnimatedBuilder( animation: controller, // ارسال کنترلر به انیمیشن بیلدر builder: (BuildContext context, Widget child) { return Container( width: animation.value, height: animation.value, child: Image.network('https://javareshkian.ir/wp-content/uploads/2020/03/javareshkian4.png') ); }, ), RaisedButton( onPressed: () { if (controller.isDismissed) // اگر انیمشن شروع نشده بود controller.forward(); // اجرای انیمیشن else if (controller.isCompleted) // اگر انیمیشن اتمام یافته بود controller.reverse(); // اجرای معکوس انیمیشن }, child: Text('شروع انیمیشن'), ) ], ), ), ); } }
خروجی کد بالا به شکل زیر است :
جهت کسب اطلاعات بیشتر از این آدرس به سایت اصلی فلاتر مراجعه نمایید .
امیدوارم این مطلب برای شما مفید باشد .