پروژه ۱ : تم Instagram
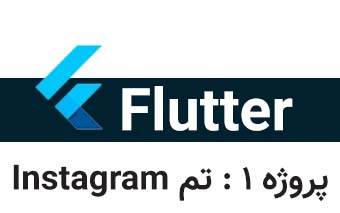
در این پروژه سعی شده با استفاده از ویجت Scaffold شکل ظاهری اپلیکیشن اینستاگرام شبیه سازی شود . توجه داشته باشید که نکات آموزشی که در این قطعه کد وجود دارد ، مد نظر بوده است نه شباهت صددرصدی ! خروجی کار شبیه تصویر زیر خواهد بود :
در ادامه کد این پروژه قابل مشاهده است :
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } // ویجت اصلی پروژه class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Instagram App', theme: new ThemeData( primaryIconTheme: IconThemeData(color: Colors.black), primaryTextTheme: TextTheme(title: TextStyle(color: Colors.black)), ), home: MyHomePage(), ); } } // به منظور پشتیبانی از رویداد تاچ کاربر از نوع استیت فول تعریف شده است class MyHomePage extends StatefulWidget { @override State<StatefulWidget> createState() { // TODO: implement createState return MyHomePageState(); } } class MyHomePageState extends State<MyHomePage> { // نگهدارنده نام صفحه در حال انتخاب String currentPageName = 'home'; // مجموعه حاوی ویجت های صفحات final Map<String, Widget> PageLoad = <String, Widget>{ 'home': new HomePage(), 'pluse': new PlusePage(), 'search': new SearchPage(), 'profile': new ProfilePage(), 'favorite': new FavoritePage() }; // تابع تغییر صفحه پس از تاچ کردن کاربر changePage(String page) { setState(() { currentPageName = page; }); } // تغییر رنگ آیکون فعال Color currentColor(String page) { return currentPageName == page ? Colors.black : Colors.blueGrey; } // سازنده ویجت AppBar final appbar = new AppBar( backgroundColor: Colors.white70, leading: IconButton( icon: Icon(Icons.camera_alt), onPressed: () { print('leading'); }, ), elevation: 1.0, title: SizedBox( height: 40.0, child: Image.network('https://javareshkian.ir/wp-content/uploads/2020/04/Instagram.png'), ), centerTitle: true, actions: <Widget>[ Padding( padding: const EdgeInsets.symmetric(horizontal: 12.0), child: IconButton( icon: Icon(Icons.send), onPressed: () { print('action1'); }, ), ), ], ); @override Widget build(BuildContext context) { return Scaffold( // فراخوانی ویجت AppBar appBar: appbar, // فراخوانی تابع انتخاب صفحه body: PageLoad[currentPageName], bottomNavigationBar: Container( height: 50.0, color: Colors.white70, child: BottomAppBar( child: Row( mainAxisAlignment: MainAxisAlignment.spaceAround, children: <Widget>[ IconButton( icon: Icon( Icons.home, color: currentColor('home'), ), onPressed: () { changePage('home'); }, ), IconButton( icon: Icon(Icons.search, color: currentColor('search')), onPressed: () { changePage('search'); }, ), IconButton( icon: Icon(Icons.add_box, color: currentColor('pluse')), onPressed: () { changePage('pluse'); }, ), IconButton( icon: Icon(Icons.favorite, color: currentColor('favorite')), onPressed: () { changePage('favorite'); }, ), IconButton( icon: Icon(Icons.account_box, color: currentColor('profile')), onPressed: () { changePage('profile'); }, ), ], ), ), ), ); } } // ویجت صفحه Home class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return new Container( color: Colors.lightGreen, child: Center( child: Text('Home Page', style: Theme.of(context).textTheme.display1,), ), ); } } // ویجت صفحه Favorite class FavoritePage extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return new Container( color: Colors.lightBlue, child: Center( child: Text('Favorite Page', style: Theme.of(context).textTheme.display1,), ), ); } } // ویجت صفحه Pluse class PlusePage extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return new Container( color: Colors.redAccent, child: Center( child: Text('Pluse Page', style: Theme.of(context).textTheme.display1,), ), ); } } // ویجت صفحه Profile class ProfilePage extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return new Container( color: Colors.red, child: Center( child: Text('Profile Page', style: Theme.of(context).textTheme.display1,), ), ); } } // ویجت صفحه Search class SearchPage extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return new Container( color: Colors.cyanAccent, child: Center( child: Text('Search Page', style: Theme.of(context).textTheme.display1,), ), ); } }
در داخل کد هر کجا که لازم بوده توضیحات مناسب درج شده است . شما فقط کافیست کد فوق را کپی کرده و در فرم main.dart قرار دهید و برنامه را اجرا نمایید تا خروجی کار را مشاهده نمایید .
امیدوارم این مطلب برای شما مفید باشد .